FASwiftUI
Easily integrate FontAwesome 5 or 6 into your SwiftUI projects. Use Font Awesome icons as text in your SwiftUI views. Supports Font Awesome Pro or Free.
(Does not currently support the Duotone style.)
Installation - Swift Package Manager
- Add the Swift package to your Xcode project
- File -> Swift Packages -> Add Package Dependency
- Enter https://github.com/mattmaddux/FASwiftUI.git
- Download Font Awesome
- Go to https://fontawesome.com/download
- Download the Pro or Free version
- Drag the following files from the download to your project:
- icons.json
AND EITHER
- Font Awesome 6 Brands-Regular-400.otf
- Font Awesome 6 [Free/Pro/Sharp]-Regular-400.otf
- Font Awesome 6 [Free/Pro/Sharp]-Solid-900.otf
- Font Awesome 6 [Pro/Sharp]-Light-300.otf (Pro Only)
OR
- Font Awesome 5 Brands-Regular-400.otf
- Font Awesome 5 [Free/Pro]-Regular-400.otf
- Font Awesome 5 [Free/Pro]-Solid-900.otf
- Font Awesome 5 Pro-Light-300.otf (Pro Only)
- Add files to target - For each of the files in the last step:
- Select the file in Project Navigator
- Open the Inspectors bar on the right and select the file inspector (first tab)
- Under Target Membership select each target you need to use FASwiftUI
- Add Fonts to Info.plist
- Open your project’s info.plist
- Right-Click in a blank area and choose “Add Row”
- Name the new entry “Fonts provided by application”
- Expand the entry by clicking the triangle to the left
- Add a new entry for each of the “otf” files you added to your project, using the full filename including the extension
- You’re done!
Usage
Use a Font Awesome icon in any view:
import SwiftUI
import FASwiftUI
struct ContentView: View {
var body: some View {
FAText(iconName: "bomb", size: 200)
}
}
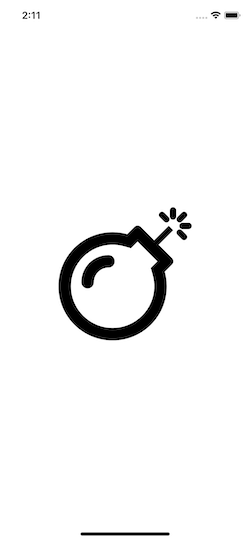
You can also choose an alternate style.
(This is ignored if the icon is a brand and currently duotone is not supported and will default back to regular.)
import SwiftUI
import FASwiftUI
struct ContentView: View {
var body: some View {
FAText(iconName: "bomb", size: 200, style: .solid)
}
}
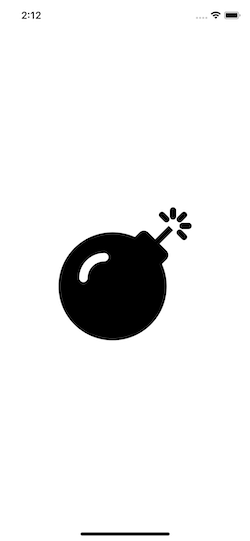
Set the color as you would any text.
import SwiftUI
import FASwiftUI
struct ContentView: View {
var body: some View {
FAText(iconName: "bomb", size: 200)
.foregroundColor(Color.red)
}
}
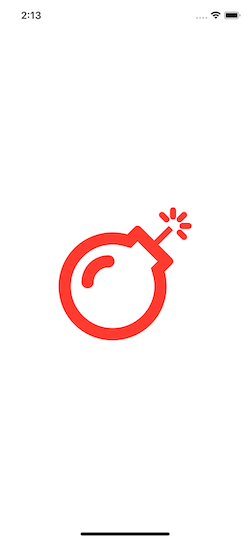